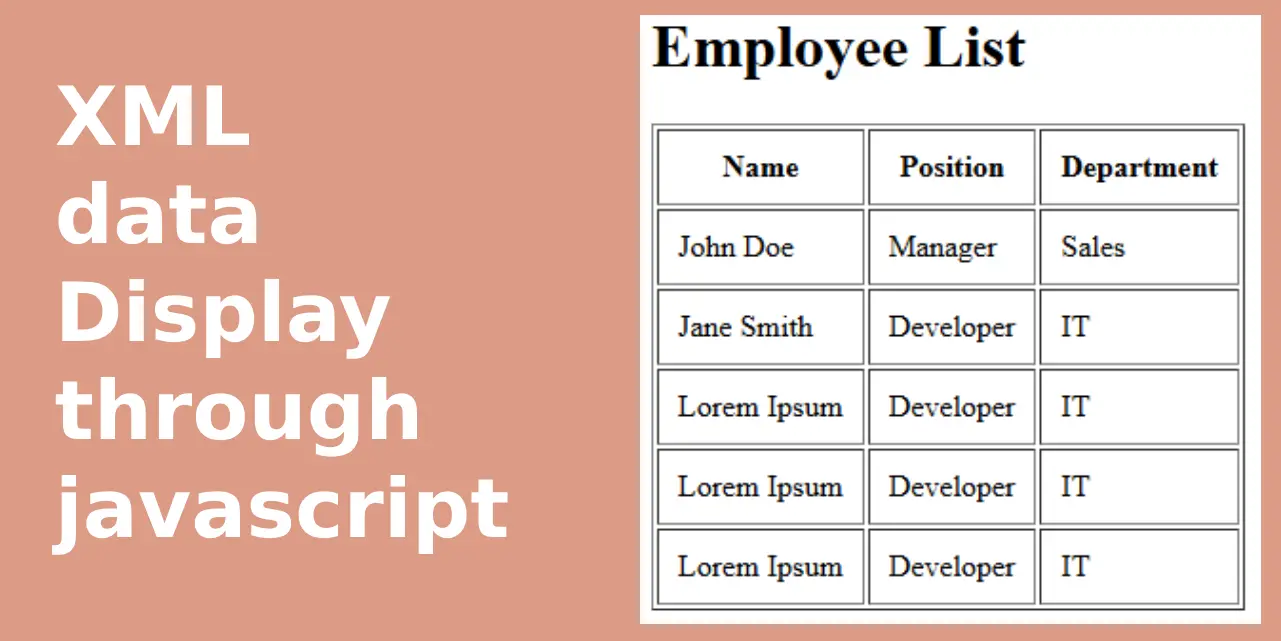
XML (Extensible Markup Language) is a versatile format that stores and organizes data in a structured manner. XML is commonly used to share data between systems because of its flexibility and readability. In web development, displaying XML data dynamically using PHP and JavaScript can enhance how users interact with content. This guide covers how to display XML data from an .xml
file using JavaScript, with an example implementation in PHP.
Understanding XML Structure
The XML file, data.xml, is structured to store employee details, making it easy to retrieve and display. Each employee has information like name, position and department. Below is a snippet of the XML structure used in this example:
Create a file where name should be data.xml
John Doe
Manager
Sales
Jane Smith
Developer
IT
Lorem Ipsum
Developer
IT
Lorem Ipsum
Developer
IT
Lorem Ipsum
Developer
IT
In this example, we will focus on how to load XML content and display it dynamically using JavaScript. The XML file contains details about employees, and we want to display this information neatly on a web page.
Displaying XML Data Using JavaScript
To load XML data, we use JavaScript’s XMLHttpRequest object, which enables us to fetch XML content asynchronously. Below is the step-by-step code used in Index.php to fetch and display XML content:
- Loading the XML File:
- The JavaScript function
loadXML()
initializes anXMLHttpRequest
to retrieve thedata.xml
file. - Once the XML file is successfully loaded, the function
displayXMLData(xmlDoc)
is called to handle and render the content.
- The JavaScript function
function loadXML() {
const xhr = new XMLHttpRequest();
xhr.open("GET", "data.xml", true);
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
const xmlDoc = xhr.responseXML;
displayXMLData(xmlDoc);
}
};
xhr.send();
}
- Parsing and Displaying the XML Content:
- Inside the
displayXMLData(xmlDoc)
function, JavaScript’sgetElementsByTagName()
method is used to retrieve employee data. - A loop iterates through each
<employee>
node, extracting thename
,position
, anddepartment
information, which is then displayed on the webpage.
- Inside the
function displayXMLData(xmlDoc) {
const employees = xmlDoc.getElementsByTagName("employee");
let output = `
Name
Position
Department
`;
for (let i = 0; i < employees.length; i++) {
const name = employees[i].getElementsByTagName("name")[0].textContent;
const position = employees[i].getElementsByTagName("position")[0].textContent;
const department = employees[i].getElementsByTagName("department")[0].textContent;
output += `
${name}
${position}
${department}
`;
}
output += "
";
document.getElementById("output").innerHTML = output;
}
// Load XML on page load
window.onload = loadXML;
- Integrating the Code in PHP:
- Although the JavaScript handles loading XML, the web page (
index.php
) serves as the container. - It includes the HTML structure and JavaScript functions required to display XML content seamlessly.
- The data is rendered in a simple list format using HTML and can be styled further using CSS for a better visual presentation.
- Although the JavaScript handles loading XML, the web page (
Benefits of Displaying XML Data
Using JavaScript to display XML data offers several advantages:
- Real-time Data: XML files can be updated dynamically, and JavaScript can fetch the latest content without refreshing the page.
- Cross-Platform Compatibility: XML is widely supported, and using JavaScript to handle XML allows developers to integrate data across any platform or device.
- Organized Data Management: XML files are easy to manage, allowing structured data management and easy debugging.
By understanding how to load XML content and display it using JavaScript, developers can create dynamic and interactive web applications. This technique is important for websites that rely on structured data such as product catalogs, employee directories, or content aggregators.
User Data Display through XML File Source Code
Send download link to: