How to Create a Dynamic Input Form Using JavaScript and PHP?
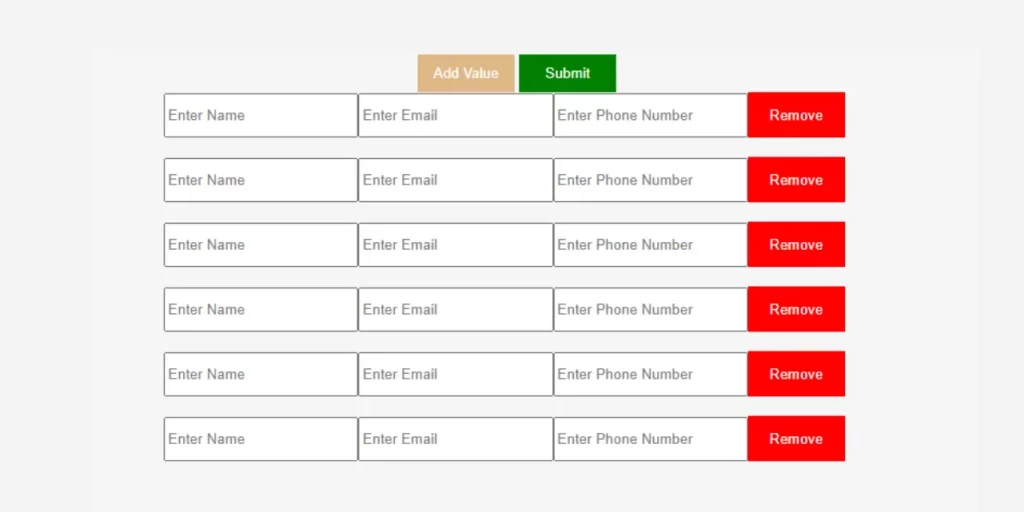
Creating dynamic input forms using JavaScript is an important part of modern web applications. They allow users to interact seamlessly by adding or removing input fields on demand. This article demonstrates how to create a dynamic form using JavaScript for the front end and PHP for backend processing with data storage in a MySQL database.
How to create dynamic input forms using JavaScript
A dynamic form enables users to create or modify form fields without reloading the page. This feature is commonly used to collect multiple entries such as name, email, and phone number, where the number of inputs may differ for each user.
In this example, we’ll implement a dynamic form that:
- Adds new Dynamic Input Form Using PHP.
- Removes unwanted input fields before submitting.
- Handles form submission in PHP and stores the data in a MySQL database.
Create dynamic input forms using JavaScript
How to Create a Dynamic Form?
- Frontend Implementation Using JavaScript
The frontend handles adding and removing input fields dynamically. JavaScript is used to dynamically create new input fields for name, email and phone and remove them as needed.
Here’s the JavaScript code:
document.getElementById("addinput").addEventListener("click", function(event) {
event.preventDefault();
addInput();
});
function addInput() {
var form = document.getElementById("myforms");
var lineBreak = document.createElement("br");
var nameInput = document.createElement("input");
nameInput.setAttribute("type", "text");
nameInput.setAttribute("placeholder", "Enter Name");
nameInput.setAttribute("name", "name[]");
nameInput.setAttribute("class", "appinputs");
nameInput.setAttribute("required", "");
var emailInput = document.createElement("input");
emailInput.setAttribute("type", "email");
emailInput.setAttribute("placeholder", "Enter Email");
emailInput.setAttribute("name", "email[]");
emailInput.setAttribute("class", "appinputs");
emailInput.setAttribute("required", "");
var phoneInput = document.createElement("input");
phoneInput.setAttribute("type", "text");
phoneInput.setAttribute("placeholder", "Enter Phone Number");
phoneInput.setAttribute("name", "phone[]");
phoneInput.setAttribute("class", "appinputs");
phoneInput.setAttribute("required", "");
var linesbreak=document.createElement("br")
var removeButton = document.createElement("input");
removeButton.setAttribute("type", "button");
removeButton.setAttribute("value", "Remove");
removeButton.setAttribute("class","appinputs removeclass");
removeButton.addEventListener("click", function(event) {
event.preventDefault();
form.removeChild(lineBreak);
form.removeChild(nameInput);
form.removeChild(emailInput);
form.removeChild(phoneInput);
form.removeChild(removeButton);
});
form.appendChild(lineBreak);
form.appendChild(nameInput);
form.appendChild(emailInput);
form.appendChild(phoneInput);
form.appendChild(removeButton);
form.appendChild(linesbreak);
}
2. Backend Implementation Using PHP
PHP handles submitted form data by sanitizing the inputs and storing them in a MySQL database. Below is the PHP code to handle the form submission:
Benefits of a Dynamic Form
Using dynamic forms offers several advantages:
- Flexibility: Allows users to input variable amounts of data.
- User-Friendly: Simplifies data entry by enabling on-the-fly customization.
- Efficient: Reduces page reloads and enhances user experience.
Database Design
The following table schema is used to store form data in a MySQL database:
CREATE TABLE dynamic_form_data (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(100),
phone VARCHAR(20)
);
Conclusion
A dynamic input forms using JavaScript built with PHP is a practical solution for efficiently collecting variable data. By leveraging JavaScript for dynamic functionality and PHP for backend processing, you can create an interactive and user-friendly form for your web application. With the addition of MySQL, the collected data can be stored securely and accessed later.
Add Dynamic Input Source Code
Send download link to: