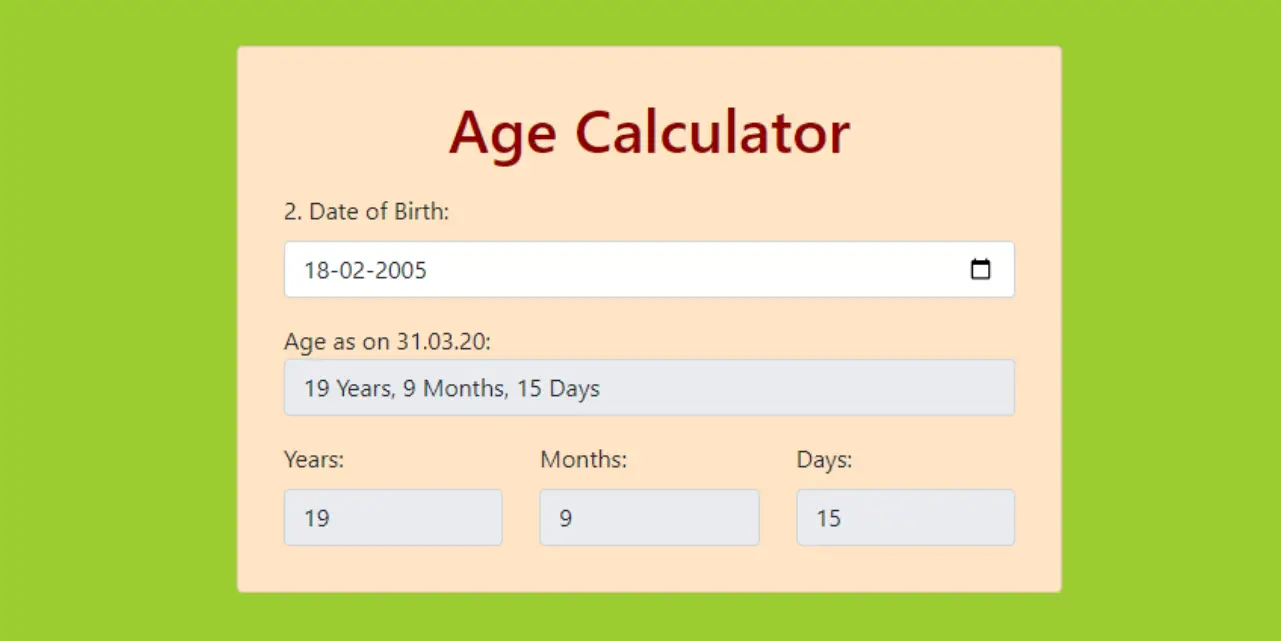
Age Calculator application calculates age based on a given date of birth, and displays the result in years, months and days. Below is an explanation of the code with details of its functionality.
Create HTML Code
The structure of the application is designed using HTML. It consists of a simple form where the user enters their date of birth, and the calculated age is displayed.
Age Calculator
Age Calculator
Create style.css
CSS code styles the background, card layout, and text. This ensures that the user interface is visually appealing and responsive.
body{
background-color: yellowgreen;
}
.card
{
padding: 30px;
margin-top: 10%;
background-color: bisque;
}
.card h1{
padding-bottom: 10px;
color: darkred;
}
Create script.js
The interactive functionality of the application is controlled using JavaScript. When the user selects a date of birth, the script calculates their age.
document.getElementById("dob").addEventListener("change", function () {
const dob = new Date(this.value);
const asOnDate = new Date();
if (dob > asOnDate) {
alert("Date of Birth cannot be after 31.03.2020.");
return;
}
let years = asOnDate.getFullYear() - dob.getFullYear();
let months = asOnDate.getMonth() - dob.getMonth();
let days = asOnDate.getDate() - dob.getDate();
if (days < 0) {
months -= 1;
days += new Date(asOnDate.getFullYear(), asOnDate.getMonth(), 0).getDate();
}
if (months < 0) {
years -= 1;
months += 12;
}
document.getElementById("years").value = years;
document.getElementById("months").value = months;
document.getElementById("days").value = days;
document.getElementById("totalAge").value = `${years} Years, ${months} Months, ${days} Days`;
});
Explanation of the Script
- Event Listener:
    Adds a change
event listener to the date input (dob
).
    Executes the age calculation when a date is selected.
2. Date Calculation Logic:
    Calculates the difference in years, months, and days.
     Adjusts for cases where the current day or month is smaller than the birth date.
3. Validation:
    Ensures the selected date is not in the future. If it is, an alert is displayed.
Output:
     Updates the corresponding fields (years
, months
, days
, and totalAge
) with the calculated values.
Key Features
- Event Listener:
- Adds a
change
event listener to the date input (dob
). - Executes the age calculation when a date is selected.
2. Date Calculation Logic:
- Calculates the difference in years, months, and days.
- Adjusts for cases where the current day or month is smaller than the birth date.
3. Validation:
Ensures the selected date is not in the future. If it is, an alert is displayed.
Output:
Updates the corresponding fields (years
, months
, days
, and totalAge
) with the calculated values.
How It Works
- Open the HTML file in a browser.
- Enter your date of birth using the date picker.
- View the calculated age in years, months, and days.
This code is simple, lightweight, and perfect for demonstrating the basics of date manipulation and user interaction in web development.
Age Calculator
Age Calculator Source Code
Send download link to:
yes
yes you can use