Complete Image Validation in PHP
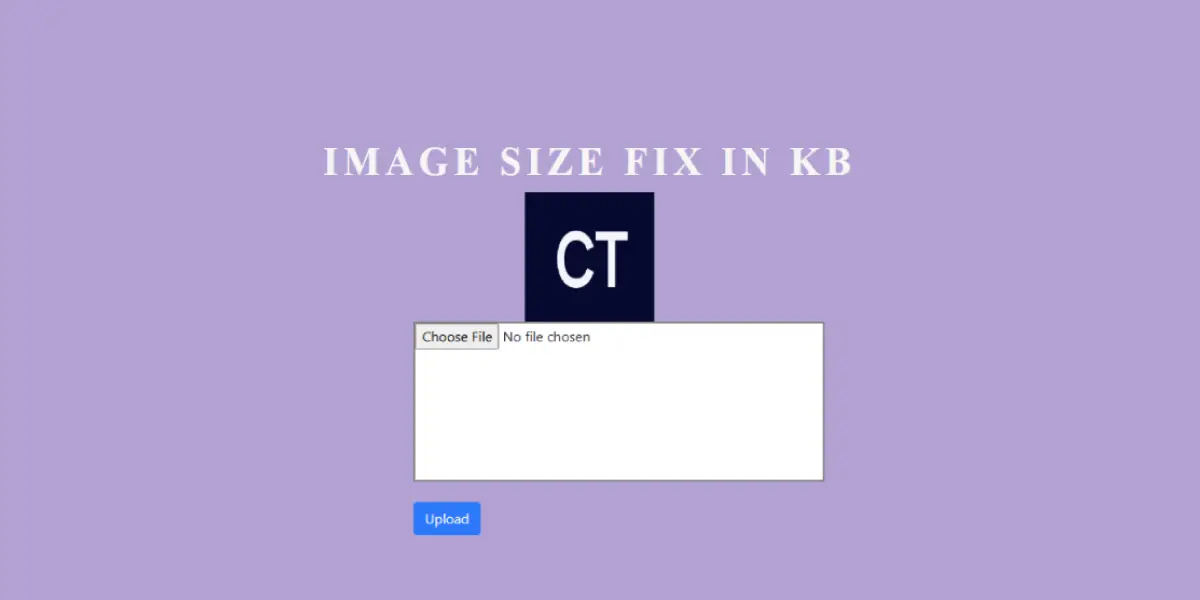
When developing web applications, it is essential to validate user-uploaded images to ensure data security, control file size, and maintain the integrity of your database. This article will walk you through a PHP script that validates image files on several important criteria like file type, size, and file upload success.
This PHP-based solution offers strong verification steps including basic security measures that prevent unauthorized file types and excessive file sizes from being uploaded. Let’s look at each verification process in detail to ensure that your image uploads are both secure and efficient.
Create HTML Form
First, create an HTML form for users to upload their images. This form uses the enctype="multipart/form-data"
attribute to handle file uploads. Here is a simple HTML structure:
Complete Image Validation | codingtutorials
Image Size fix in KB
Create Style.css page
body {
background-color: #b1a2d3;
}
h1 {
color: whitesmoke;
font-family: 'Russo One', sans-serif;
text-transform: uppercase;
text-shadow: 3px 3px #b1a2d3;
font-size: 45px;
font-weight: 600;
letter-spacing: 6px;
margin-top: 10%;
}
input[type="file"] {
width: 35%;
border: 2px solid grey;
height: 185px;
background-color: white;
}
This form includes an input
field where users can select an image file, along with a submit button to upload the file. The POST
method and enctype="multipart/form-data"
ensure the server processes the uploaded file correctly.
PHP Script to Validate the Image
The core PHP script handles file validation. Here are the details of the main validation checks:
- Check if the File was Uploaded Properly : PHP’s $_FILES superglobal contains information about the uploaded file. First, check if the form was submitted and if the file upload is error free.
session_start();
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$filetmp = $_FILES["image"]["tmp_name"];
$filename = $_FILES["image"]["name"];
$filetype = $_FILES["image"]["type"];
$filesize = $_FILES["image"]["size"];
}
2. File Type Validation : Restrict file types to avoid potential security risks. Allow only JPEG, PNG and WebP formats by checking the MIME type of the file.
$allowed_types = ["image/jpeg", "image/png", "image/webp"];
if (!in_array($filetype, $allowed_types)) {
echo "Only JPEG, PNG, and WEBP files are allowed.
";
exit;
}
3. File Size Validation : Prevent extremely large files from being uploaded. Here, we limit the file size to 50 KB.
if ($filesize > 50000) {
echo "Image size is greater than 50KB
";
exit;
}
4. File Upload and Database Storage : After passing verification, move the uploaded file to a specified folder and save the file information to the database.
$filepath = "image/" . $filename;
if (move_uploaded_file($filetmp, $filepath)) {
// Database connection
$conn = mysqli_connect("localhost", "root", "", "registrationform");
$sql = "INSERT INTO imageuploadkb (filename, filesize) VALUES ('$filename', '$filesize')";
mysqli_query($conn, $sql);
// Display the uploaded image
echo '
';
} else {
echo "File upload failed.
";
}
Explanation of Each Validation Step
- File Type Verification: By using MIME types, we tightly control the types of files that can be uploaded, which helps prevent security threats associated with unauthorized file types.
- File Size Limitation: This step ensures that large files do not consume unnecessary storage or bandwidth and provides better control over server resources.
- File Move and Database Entry: Only files that pass the above checks are moved to the desired location. We also store file metadata in the database, which is useful for later retrieval or display.
Complete PHP Code Example
Here is the complete PHP code that implements all the steps:
Only JPEG, PNG, and WEBP files are allowed.";
} elseif ($filesize > 50000) {
echo "Image size is greater than 50KB
";
} else {
$filepath = "image/" . $filename;
if (move_uploaded_file($filetmp, $filepath)) {
$conn = mysqli_connect("localhost", "root", "", "registrationform");
$sql = "INSERT INTO imageuploadkb (filename, filesize) VALUES ('$filename', '$filesize')";
mysqli_query($conn, $sql);
echo '
';
} else {
echo "File upload failed.
";
}
}
}
?>
PHP Complete Image Validation Source Code
Send download link to: