Creating an Effective Newsletter Subscription Form with Email Validation
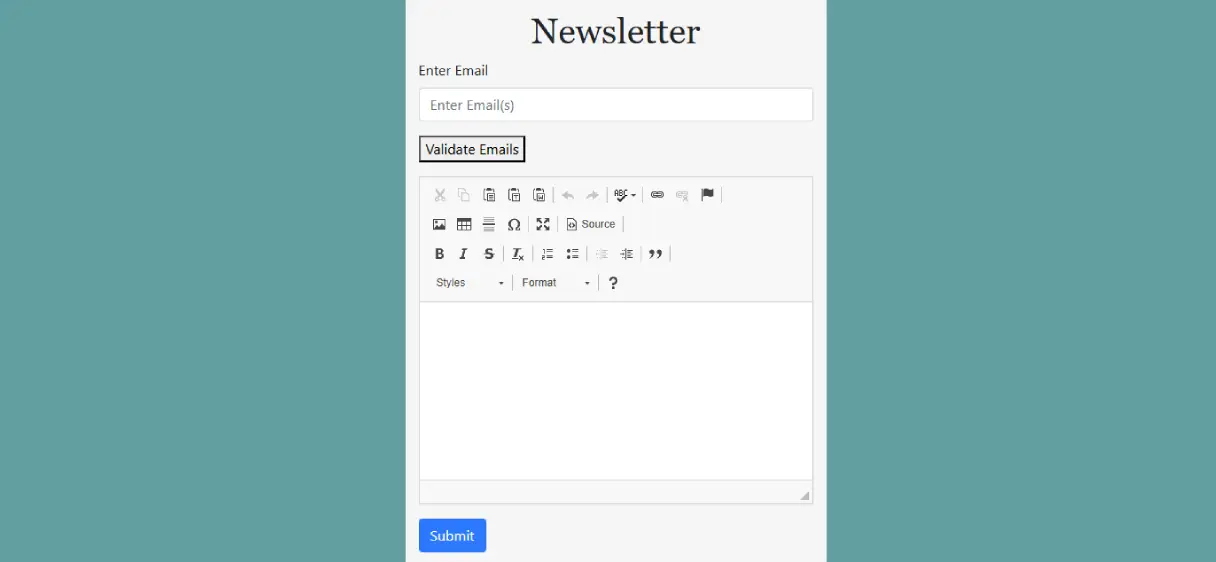
For developers building websites, integrating a user-friendly and efficient newsletter subscription form can greatly enhance user engagement and increase traffic. In this post, we’ll explore how to create a simple and clean newsletter form with a built-in email validation function using HTML, CSS, JavaScript, and CKEditor for rich text editing.
Creating the Form
Our form will consist of two main elements: an email input field for entering multiple email addresses and a text area for writing the newsletter content. To ensure that the form works flawlessly, we will implement validation for the email fields to check that the emails entered are in the correct format.
HTML Form
Newsletter
This simple structure includes:
- An input field for multiple email addresses.
- A text area for writing the newsletter message.
- A submit button to send the form data.
Form with CSS
A clean and visually appealing appearance can have a significant impact on the user experience. The CSS below styles the background, form container, and typography:
body {
background-color: cadetblue;
}
.cke_notifications_area {
display: none;
}
h1 {
font-family: Georgia, serif !important;
text-align: center;
}
.container {
margin-top: 0%;
}
.col-lg-5 {
background-color: whitesmoke;
padding: 15px;
}
The background color cadetblue
contrasts well with the whitesmoke form container, creating a clean and modern look.
Using CKEditor for Rich Text Editing
CKEditor is integrated to provide a rich text editing experience for writing newsletter messages. The following script enables CKEditor on a text area:
With CKEditor, users can add formatting like bold, italics, and links to their newsletters, giving messages a professional look.
Validating Multiple Email Addresses with JavaScript
One of the most important aspects of the form is validating the email address. We want to make sure that all emails entered are valid before submitting the form. Here is the JavaScript function to verify multiple emails:
This function checks each email against regular expression patterns for validity. If an email is invalid, the user receives feedback listing the problematic emails.
Input Validation
To make sure all fields are filled before submitting, we use a final validation check:
function Myfunction() {
var emailInput = document.getElementById("emailInput").value;
var newsletter = document.getElementById("newsletter").value;
if (emailInput === "") {
alert("Please Fill Email");
return false;
} else if (newsletter === "") {
alert("Please Fill Subject");
return false;
} else {
return true;
}
}
This ensures that neither the email field nor the message box is left blank before submitting.
Newsletter Zip File
Send download link to: