Fetch and Display User Data from an API with JavaScript
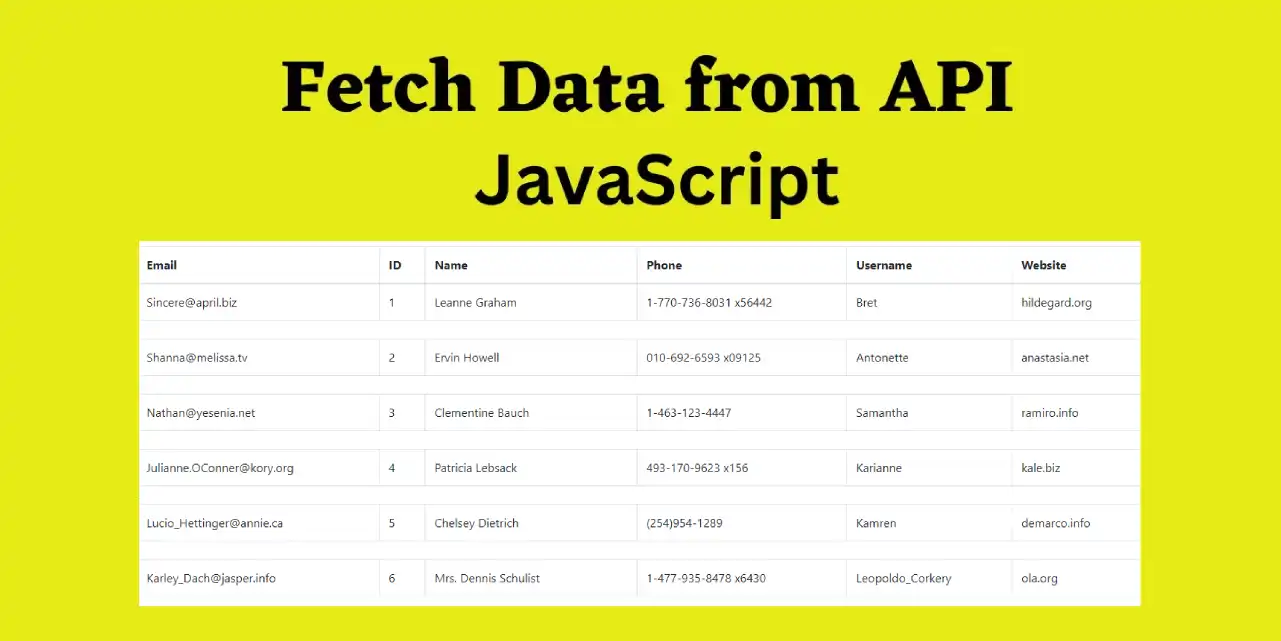
In today’s digital landscape, it is essential to manage data efficiently to provide seamless user experience on websites. A popular way to dynamically display user information is to retrieve data from an API and present it directly on a web page. This guide shows how to accomplish this using JavaScript and the Fetch API.
Understanding APIs and their role in web development
An API (Application Programming Interface) allows different software applications to communicate with each other and share data. In the context of web development, APIs provide an efficient way to retrieve data from a server without reloading the web page. For example, a user can provide details such as name, email, and other profile data to the Information API. This approach saves development time and simplifies data management across different platforms.
For this example, we’ll use the JSONPlaceholder API, which is a free online service that provides a series of dummy data for testing and learning purposes. Specifically, we will get user data from this API, including details like user ID, name, email, and website.
Step-by-Step Guide to Fetching and Displaying Data with JavaScript
Set Up the HTML Table Structure :
To display user data, create an HTML table with headers such as “Email,” “ID,” “Name,” etc. Each column will represent a specific attribute of the user data. The use of table format allows for an organized view and user-friendly interface.
Email
ID
Name
Phone
Username
Website
Write JavaScript Code to Fetch Data :
To fetch data from an API, JavaScript’s Fetch API can be used. This API enables us to send HTTP requests and receive responses asynchronously, meaning there is no need to reload the page while waiting for data. Here is the code to fetch and display user data:
async function callApi() {
try {
let result = await fetch('https://jsonplaceholder.typicode.com/users');
result = await result.json();
document.getElementById("userdata").innerHTML = result.map(user =>
`
${user.email}
${user.id}
${user.name}
${user.phone}
${user.username}
${user.website}
`
).join('');
} catch (error) {
console.error("Error fetching data: ", error);
}
}
callApi();
Here are the details of the code:
await fetch(...)
: Fetches data from the specified API.result.json()
: Converts the raw data into a JavaScript object.result.map(...)
: Maps each user object to a new HTML table row.
Testing and Refinement
It is necessary to test the code to make sure everything displays correctly. Open the page in a browser, and you should see the user data loaded into the table. Using browser developer tools, you can verify that the data was received properly and troubleshoot any issues.
Conclusion
Using JavaScript to fetch and display API data is an efficient approach for developers who want to keep content dynamic and relevant. This method ensures that data is up to date and reduces the load on the server, making it a scalable solution. For any developer who wants to improve the interactivity and SEO potential of their website, mastering API data fetching is a highly valuable skill.
Fetch Data Api in Javascript Source Code
Send download link to: