How to Create an Amazing Wall Clock in JavaScript and HTML
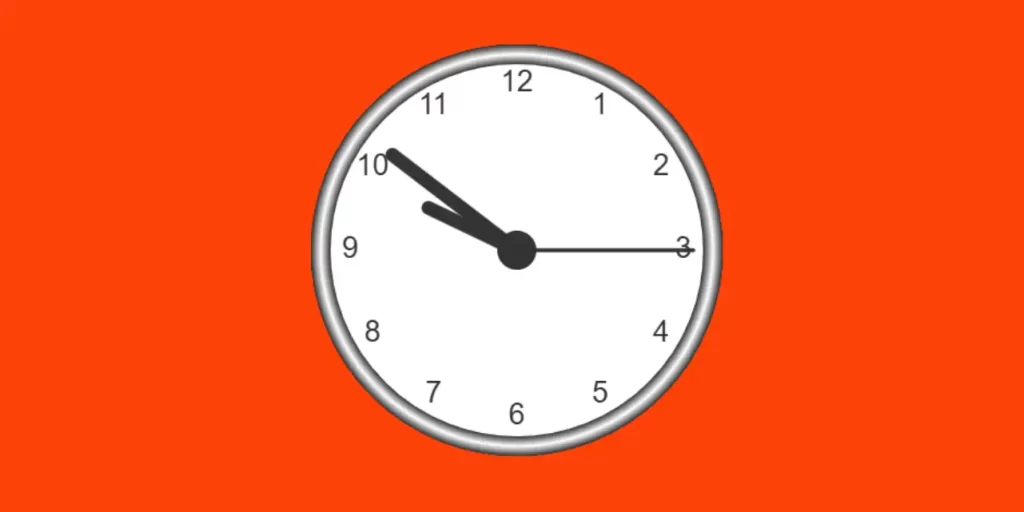
Wall clocks aren’t just useful; They can also enhance the visible appeal of a website or mission. Creating a wall clock in JavaScript using the HTML5 canvas detail to render photographs is a extremely good way to enhance your programming competencies. In this article, we can guide you in developing a dynamic and interactive clock the usage of JavaScript.
What You'll Learn:
- How to use the HTML5 canvas element.
- How to create shapes and text on canvas using JavaScript.
- Implementing real time clock functionality.
Creating a Wall Clock in JavaScript Step by Step Guide
Step 1: Create HTML Structure
The first step is to create a simple HTML structure for your clock. Use the following code to set a canvas element, which will act as the clock’s surface.
Wall Clock in JavaScript
Step 2: Creating JavaScript for the clock.
The main functionality of the wall clock is implemented in JavaScript. The code to create the clock face, numbers and hands is given below:
const canvas = document.getElementById("canvas");
const ctx = canvas.getContext("2d");
let radius = canvas.height / 2;
ctx.translate(radius, radius);
radius = radius * 0.90;
setInterval(drawClock, 1000);
function drawClock() {
drawFace(ctx, radius);
drawNumbers(ctx, radius);
drawTime(ctx, radius);
}
function drawFace(ctx, radius) {
const grad = ctx.createRadialGradient(0, 0, radius * 0.95, 0, 0, radius * 1.05);
grad.addColorStop(0, '#333');
grad.addColorStop(0.5, 'white');
grad.addColorStop(1, '#333');
ctx.beginPath();
ctx.arc(0, 0, radius, 0, 2 * Math.PI);
ctx.fillStyle = 'white';
ctx.fill();
ctx.strokeStyle = grad;
ctx.lineWidth = radius * 0.1;
ctx.stroke();
ctx.beginPath();
ctx.arc(0, 0, radius * 0.1, 0, 2 * Math.PI);
ctx.fillStyle = '#333';
ctx.fill();
}
function drawNumbers(ctx, radius) {
ctx.font = radius * 0.15 + "px arial";
ctx.textBaseline = "middle";
ctx.textAlign = "center";
for (let num = 1; num < 13; num++) {
let ang = num * Math.PI / 6;
ctx.rotate(ang);
ctx.translate(0, -radius * 0.85);
ctx.rotate(-ang);
ctx.fillText(num.toString(), 0, 0);
ctx.rotate(ang);
ctx.translate(0, radius * 0.85);
ctx.rotate(-ang);
}
}
function drawTime(ctx, radius) {
const now = new Date();
let hour = now.getHours();
let minute = now.getMinutes();
let second = now.getSeconds();
// Hour
hour = hour % 12;
hour = (hour * Math.PI / 6) + (minute * Math.PI / (6 * 60)) + (second * Math.PI / (360 * 60));
drawHand(ctx, hour, radius * 0.5, radius * 0.07);
// Minute
minute = (minute * Math.PI / 30) + (second * Math.PI / (30 * 60));
drawHand(ctx, minute, radius * 0.8, radius * 0.07);
// Second
second = (second * Math.PI / 30);
drawHand(ctx, second, radius * 0.9, radius * 0.02);
}
function drawHand(ctx, pos, length, width) {
ctx.beginPath();
ctx.lineWidth = width;
ctx.lineCap = "round";
ctx.moveTo(0, 0);
ctx.rotate(pos);
ctx.lineTo(0, -length);
ctx.stroke();
ctx.rotate(-pos);
}
Conclusion
Creating a wall clock in JavaScript is a outstanding way to beautify your coding talents whilst including a dynamic element for your website. With HTML5 Canvas and JavaScript, you may create a beautiful clock that updates in real time. Start experimenting today and make your undertaking specific!
Frequently Asked Questions
1.Can I customize watch colors and styles? Yes, you can modify canvas styles, gradients, and fonts in JavaScript code to suit your preferences.
2. Is this clock responsive? Although this example isn’t fully responsive, you could adjust the canvas dimensions and use CSS to evolve it to unique display screen sizes.
3. Which browsers support the canvas element? HTML5 Canvas is supported by means of all modern-day browsers along with Chrome, Firefox, Safari, and Edge.
Javascript Clock Source Code
Send download link to: