Complete Sign-Up Form Using PHP, Bootstrap, and jQuery
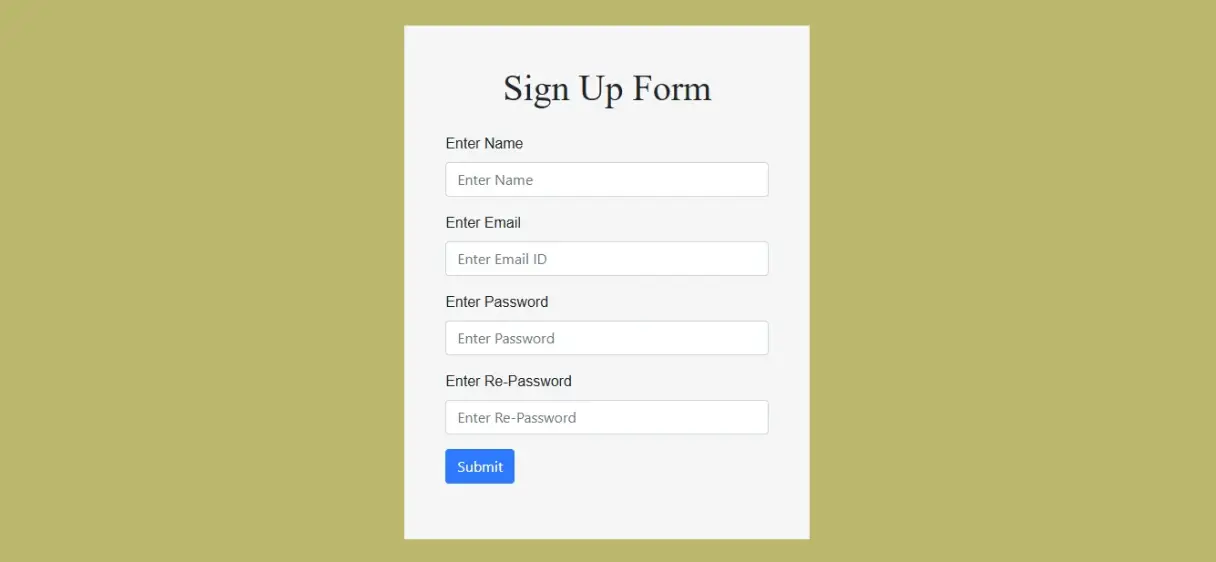
In this post, we’ll learn how to create a fully functional sign-up form using PHP for the backend, Bootstrap for styling, and JavaScript for front-end validation.
Features of the Sign-Up Form:
- Responsive Design with Bootstrap: The form is styled using Bootstrap 4, ensuring a clean and responsive interface.
- Data Validation: Both client-side (JavaScript) and server-side (PHP) validation are used to enhance security and ensure the form is properly filled.
- Database Integration: User details like name, email, and password are stored in a MySQL database after validation.
- Email Confirmation: Once a user successfully signs up, a confirmation email is sent.
Before getting started, ensure that you have a basic understanding of HTML, PHP, and MySQL. You’ll also need a working server environment with access to a MySQL database.
HTML Structure
We start to creating the HTML structure of the sign-up form. Bootstrap is used to ensure that the form looks professional and is responsive on different devices.
console.log( 'Code is Poetry' );
Sign Up Form
Adding Validation with JavaScript
For better user experience, we validate form inputs on the client side before sending the data to the server. This will ensure that required fields are not left blank and both passwords match.
Backend Processing with PHP and MySQL
Now, we will create a PHP script (process_signup.php) that processes the form data. The script will check if the email already exists in the database and if not, insert the user’s details into the sign table.
Email already exists!";
} else {
// Insert new user into the database
$sql = "INSERT INTO sign (name, email, password) VALUES ('$name', '$email', '$password')";
if(mysqli_query($conn, $sql)) {
// Send confirmation email
$to = $email;
$subject = "Welcome to Our Website!";
$message = "Hi $name,\n\nThank you for signing up!";
$headers = "From: no-reply@yourwebsite.com";
if(mail($to, $subject, $message, $headers)) {
echo "Confirmation email sent!
";
} else {
echo "Failed to send confirmation email.
";
}
} else {
echo "Error registering user.
";
}
}
}
?>
Create the conn.php File for the MySQLi Connection
In this file, we’ll establish the connection to the MySQL database.
In the above code:
"localhost"
is the server (usually localhost when working on a local server)."root"
is the MySQL username (default for local environments).""
is the MySQL password (leave empty if there is no password for the root user)."signup_form"
is the name of the database where you are storing the user information.
Once the code is implemented, you can test it on your local or live server. Make sure the database connection is correct, and the form data is being entered properly. Check the email confirmation functionality to see if users are receiving welcome emails.
Php complete form
Send download link to:
This blog is very helpful for me for design a Sign-Up Form. Thanku So much