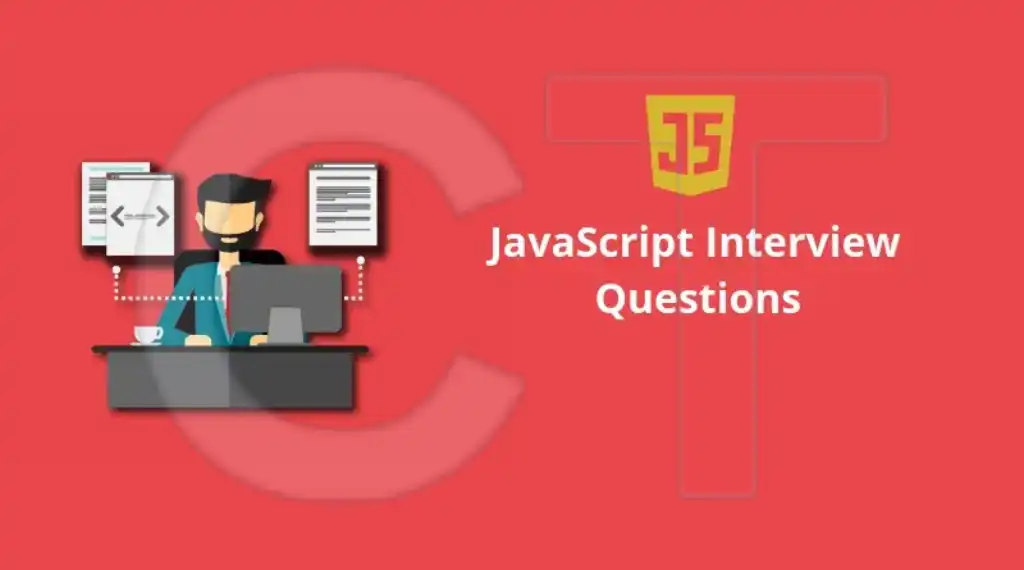
What is JavaScript?
JavaScript is a high-level, interpreted programming language used to create dynamic and interactive web content.
What are the data types supported by JavaScript?
JavaScript supports the following data types:
- Primitive:
string
,number
,boolean
,null
,undefined
,symbol
,bigint
- Non-Primitive:
object
What is the difference between var, let, and const?
var
: Scope can be re-declared and hoisted.let
: Block-scoped, can’t be re-declared, can’t be hoisted.const
: Block-scoped, immutable, not hoisted.
What is the difference between == and ===?
==
: Compares values after type conversion (loose equality).===
: Compares values without type conversion (strict equality).
What is the purpose of the isNaN() function?
The isNaN()
function checks whether a value is NaN
(Not-a-Number).
What is the difference between null and undefined?
null
: Represents the intentional absence of a value.undefined
: Represents a variable that has been declared but not assigned a value.
Explain hoisting in JavaScript.
Hoisting is JavaScript’s behavior of moving declarations (var
, function
) to the top of their scope during compilation.
What are JavaScript closures?
A closure is a function that has access to its outer function’s variables even after the outer function has executed.
What is the difference between function declarations and function expressions?
- Function Declaration: Defined with the
function
keyword and hoisted. - Function Expression: Assigned to a variable and not hoisted.
What are JavaScript promises?
Promises are used to handle asynchronous operations. They can be in one of three states: pending
, fulfilled
, or rejected
.
What is the difference between call(), apply(), and bind()?
call()
: Invokes a function with a specifiedthis
context and arguments.apply()
: Similar tocall()
, but takes arguments as an array.bind()
: Returns a new function with the specifiedthis
context.
What is the event loop in JavaScript?
The event loop is a mechanism that ensures non-blocking execution by handling asynchronous operations and executing callbacks.
What are higher-order functions?
A higher-order function is a function that takes another function as an argument or returns a function.
What is the difference between map(), filter(), and reduce()?
map()
: Creates a new array by applying a function to each element.filter()
: Creates a new array with elements that pass a condition.reduce()
: Reduces an array to a single value by applying a function.
Explain the this keyword in JavaScript.
The value of this
depends on how a function is called:
- In a method,
this
refers to the object. - In a regular function,
this
refers to the global object (window
in browsers).
What is the difference between synchronous and asynchronous JavaScript?
- Synchronous: Executes code sequentially, blocking further execution.
- Asynchronous: Executes code without blocking, using callbacks, promises, or
async/await
.
What is the purpose of async and await in JavaScript?
async
functions return promises. await
pauses execution until the promise is resolved.
What is destructuring in JavaScript?
Destructuring is a syntax for extracting values ​​from arrays or objects:
const {name, age} = person;
const [first, second] = array;
What are template literals in JavaScript?
Templates allow to embed variables and expressions using literal backticks () and ${}` syntax:
const greeting = `Hello, ${name}!`;
What is the difference between undefined and not defined in JavaScript?
undefined
: A variable is declared but not assigned a value.not defined
: A variable is not declared in the program.
What is the prototype in JavaScript?
Prototype
is an object associated with every function and object, which is used for inheritance.
What is the difference between setTimeout and setInterval?
setTimeout
: Executes a function after a delay.setInterval
: Executes a function repeatedly at a specified interval.